https://www.acmicpc.net/problem/10866
10866번: 덱
첫째 줄에 주어지는 명령의 수 N (1 ≤ N ≤ 10,000)이 주어진다. 둘째 줄부터 N개의 줄에는 명령이 하나씩 주어진다. 주어지는 정수는 1보다 크거나 같고, 100,000보다 작거나 같다. 문제에 나와있지
www.acmicpc.net
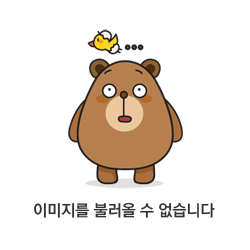
Deque, LinkedList 사용
import java.io.*;
import java.util.Deque;
import java.util.LinkedList;
import java.util.StringTokenizer;
public class Main {
static Deque<Integer> deque = new LinkedList<>();
static StringTokenizer st;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int N = Integer.parseInt(br.readLine());
for(int i=0; i<N; i++){
st= new StringTokenizer(br.readLine());
executeCommand();
}
}
public static void executeCommand(){
switch (st.nextToken()){
case "push_front":
push_front();
break;
case "push_back":
push_back();
break;
case "pop_front" :
pop_front();
break;
case "pop_back":
pop_back();
break;
case "size":
size();
break;
case "empty":
empty();
break;
case "front":
front();
break;
case "back":
back();
break;
}
}
public static void push_front(){
deque.offerFirst(Integer.parseInt(st.nextToken()));
}
public static void push_back(){
deque.offerLast(Integer.parseInt(st.nextToken()));
}
public static void pop_front(){
if(deque.isEmpty()){
System.out.println(-1);
} else{
System.out.println(deque.pollFirst());
}
}
public static void pop_back() {
if(deque.isEmpty()){
System.out.println(-1);
} else{
System.out.println(deque.pollLast());
}
}
public static void size(){
System.out.println(deque.size());
}
public static void empty() {
if(deque.isEmpty()){
System.out.println(1);
}else{
System.out.println(0);
}
}
public static void front(){
if(deque.isEmpty()){
System.out.println(-1);
}else{
System.out.println(deque.peekFirst());
}
}
public static void back(){
if(deque.isEmpty()){
System.out.println(-1);
}else{
System.out.println(deque.peekLast());
}
}
}
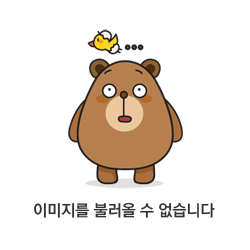
구현체에 ArrayDeque,ConcurrentLinkedDeque, LinkedBlockingDeque, LinkedList 등이 있다고 한다.
'알고리즘 > 백준' 카테고리의 다른 글
백준 17413번 - 단어 뒤집기 2(Java 8) (0) | 2022.03.11 |
---|---|
백준 1406번 - 에디터 (Java 8) (0) | 2022.03.11 |
백준 10845번 - 큐 (Java 8) (0) | 2022.03.10 |
백준 1158번 - 요세푸스 (Java 8) (0) | 2022.03.10 |
백준 1874번 - 스택 수열 (Java 8) (0) | 2022.03.09 |
댓글